Netty代码写法总结4Java
文章发布较早,内容可能过时,阅读注意甄别。
# 总结4
# Final类并在构造函数里验证空参
public final class ProxyConnectionEvent {
private final String protocol;
private final String authScheme;
private final SocketAddress proxyAddress;
private final SocketAddress destinationAddress;
private String strVal;
/**
* Creates a new event that indicates a successful connection attempt to the destination address.
*/
public ProxyConnectionEvent(
String protocol, String authScheme, SocketAddress proxyAddress, SocketAddress destinationAddress) {
if (protocol == null) {
throw new NullPointerException("protocol");
}
if (authScheme == null) {
throw new NullPointerException("authScheme");
}
if (proxyAddress == null) {
throw new NullPointerException("proxyAddress");
}
if (destinationAddress == null) {
throw new NullPointerException("destinationAddress");
}
this.protocol = protocol;
this.authScheme = authScheme;
this.proxyAddress = proxyAddress;
this.destinationAddress = destinationAddress;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
# Epoll调用原生方法
/**
* Tells if <a href="http://netty.io/wiki/native-transports.html">{@code netty-transport-native-epoll}</a> is supported.
*/
public final class Epoll {
private static final Throwable UNAVAILABILITY_CAUSE;
static {
Throwable cause = null;
int epollFd = -1;
int eventFd = -1;
try {
epollFd = Native.epollCreate();
eventFd = Native.eventFd();
} catch (Throwable t) {
cause = t;
} finally {
if (epollFd != -1) {
try {
Native.close(epollFd);
} catch (Exception ignore) {
// ignore
}
}
if (eventFd != -1) {
try {
Native.close(eventFd);
} catch (Exception ignore) {
// ignore
}
}
}
if (cause != null) {
UNAVAILABILITY_CAUSE = cause;
} else {
UNAVAILABILITY_CAUSE = null;
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
原生方法:
final class Native {
static {
String name = SystemPropertyUtil.get("os.name").toLowerCase(Locale.UK).trim();
if (!name.startsWith("linux")) {
throw new IllegalStateException("Only supported on Linux");
}
NativeLibraryLoader.load("netty-transport-native-epoll", PlatformDependent.getClassLoader(Native.class));
}
public static native int eventFd();
public static native void eventFdWrite(int fd, long value);
public static native void eventFdRead(int fd);
public static native int epollCreate();
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
# 自定义实现集合,并可以比较
public interface ChannelGroup extends Set<Channel>, Comparable<ChannelGroup> {
/**
* Returns the name of this group. A group name is purely for helping
* you to distinguish one group from others.
*/
String name();
/**
* Returns the {@link Channel} which has the specified {@link ChannelId}.
*
* @return the matching {@link Channel} if found. {@code null} otherwise.
*/
Channel find(ChannelId id);
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
2
3
4
5
6
7
8
9
10
11
12
13
14
15
# 自定义迭代器
/**
* {@link ChannelException} which holds {@link ChannelFuture}s that failed because of an error.
*/
public class ChannelGroupException extends ChannelException implements Iterable<Map.Entry<Channel, Throwable>> {
private static final long serialVersionUID = -4093064295562629453L;
private final Collection<Map.Entry<Channel, Throwable>> failed;
public ChannelGroupException(Collection<Map.Entry<Channel, Throwable>> causes) {
if (causes == null) {
throw new NullPointerException("causes");
}
if (causes.isEmpty()) {
throw new IllegalArgumentException("causes must be non empty");
}
failed = Collections.unmodifiableCollection(causes);
}
/**
* Returns a {@link Iterator} which contains all the {@link Throwable} that was a cause of the failure and the
* related id of the {@link Channel}.
*/
@Override
public Iterator<Map.Entry<Channel, Throwable>> iterator() {
return failed.iterator();
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
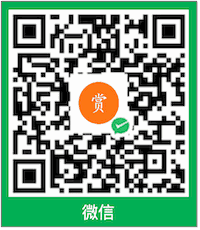
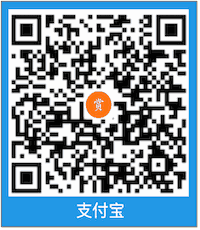
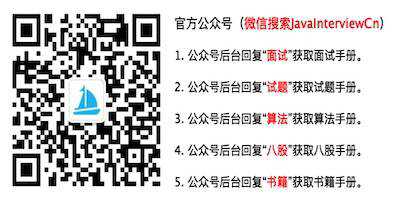