尽量减少恶意软件的传播Java
文章发布较早,内容可能过时,阅读注意甄别。
# 题目
给出了一个由 n 个节点组成的网络,用 n × n 个邻接矩阵图 graph 表示。在节点网络中,当 graph[i][j] = 1 时,表示节点 i 能够直接连接到另一个节点 j。
一些节点 initial 最初被恶意软件感染。只要两个节点直接连接,且其中至少一个节点受到恶意软件的感染,那么两个节点都将被恶意软件感染。这种恶意软件的传播将继续,直到没有更多的节点可以被这种方式感染。
假设 M(initial) 是在恶意软件停止传播之后,整个网络中感染恶意软件的最终节点数。
如果从 initial 中移除某一节点能够最小化 M(initial), 返回该节点。如果有多个节点满足条件,就返回索引最小的节点。
请注意,如果某个节点已从受感染节点的列表 initial 中删除,它以后仍有可能因恶意软件传播而受到感染。
示例 1:
输入:graph = [[1,1,0],[1,1,0],[0,0,1]], initial = [0,1]
输出:0
示例 2:
输入:graph = [[1,0,0],[0,1,0],[0,0,1]], initial = [0,2]
输出:0
示例 3:
输入:graph = [[1,1,1],[1,1,1],[1,1,1]], initial = [1,2]
输出:1
提示:
- n == graph.length
- n == graph[i].length
- 2 <= n <= 300
- graph[i][j] == 0 或 1.
- graph[i][j] == graph[j][i]
- graph[i][i] == 1
- 1 <= initial.length <= n
- 0 <= initial[i] <= n - 1
- initial 中所有整数均不重复
# 思路
// 只要是一个连通图,里面有两个恶意节点,删去哪一个都是不行的,所以要找到单个恶意节点的连通图,如果都没有,那就返回所有恶意节点中里面最小的节点值。
# 解法
class Solution {
// 只要是一个连通图,里面有两个恶意节点,删去哪一个都是不行的,所以要找到单个恶意节点的连通图,如果都没有,那就返回所有恶意节点中里面最小的节点值。
public int minMalwareSpread(int[][] graph, int[] initial) {
int node = 1000;
// 只要是连通图,如果污染节点大于2个那么整个连通图都会被污染
// 所以要找到单个污染节点的连通图,如果都没有,那就返回最小的节点值
boolean[] visited = new boolean[graph.length];
HashSet<Integer> set = new HashSet<>();
for (int i : initial) {
set.add(i);
node = Math.min(i, node);
}
Queue<Integer> queue = new ArrayDeque<>();
PriorityQueue<Integer> priorityQueue = new PriorityQueue<>();
int count, max_count = -1;
// 划分连通区域
for (int i = 0; i < visited.length; i++) {
if (visited[i]) continue;
queue.add(i);
count = 1;
visited[i] = true;
while (!queue.isEmpty()) {
Integer poll = queue.poll();
if (set.contains(poll)) {
priorityQueue.add(poll);
}
for (int j = 0; j < graph[poll].length; j++) {
if (graph[poll][j] == 0 || visited[j]) continue;
queue.add(j);
count++;
visited[j] = true;
}
}
if (priorityQueue.size() == 1) {
if (max_count == count) {
node = Math.min(node, priorityQueue.poll());
}
if (max_count < count) {
max_count = count;
node = priorityQueue.poll();
}
}
priorityQueue.clear();
}
return node;
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
# 总结
- 分析出几种情况,然后分别对各个情况实现
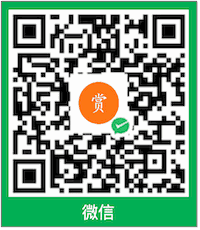
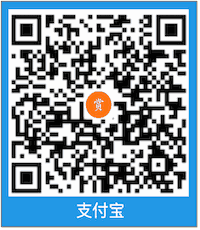
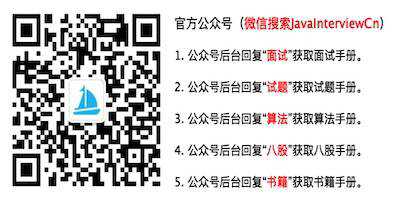